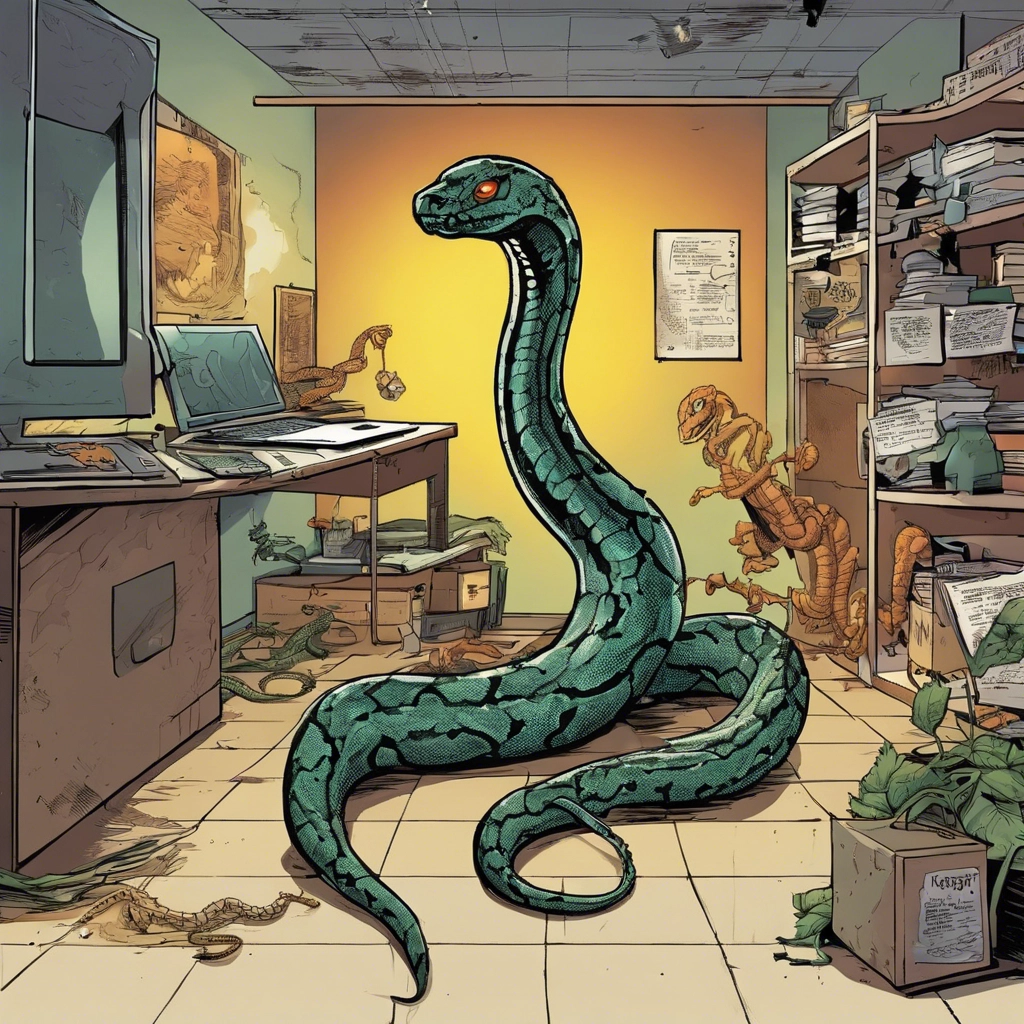
Introduction: Scorpion Vision offers a sophisticated API that allows users to interact with and automate various aspects of their vision system. One of the central features is the ability to manage and manipulate the tools responsible for image analysis using the ToolList
object. This functionality is particularly powerful when integrated with Python scripts.
GetToolList(): At the heart of tool management in Scorpion Vision is the GetToolList()
function. This function retrieves the ToolList
instance, which is the collection of tools currently loaded in Scorpion Vision. It can target different levels within the toolbox hierarchy:
GetToolList()
: Retrieves theToolList
at the current level.GetToolList('.')
: Same asGetToolList()
.GetToolList('..')
: Accesses the toolbox’s parentToolList
.GetToolList('TB')
: Gets theToolList
of the toolbox named ‘TB’ at the current level.GetToolList(':')
: Fetches the root levelToolList
, no matter the current position.GetToolList(':TB')
: Obtains theToolList
of the toolbox ‘TB’ at the root level from any level.
Attributes and Methods: The ToolList
object comes with a suite of attributes and methods allowing comprehensive control over your tools:
count
: (Read-Only) Gets the number of tools in theToolList
.next
: (Read-Write) Sets or gets the name of the next tool to execute.recursiveCount
: (Read-Only) Checks the current recursive counter to avoid entering too many recursive calls.execCount
: (Read-Only) Obtains the total number of tools executed in the last Inspect command.config
: (Read-Write) Interacts with the toolbox configuration XML string.
You can add, insert, delete, load, save, and manipulate the execution order of the tools using methods like add()
, insert()
, delete()
, etc.
Code Examples: Let’s walk through some practical examples of how to use the ToolList
object within Scorpion Vision:
Example 1 – Execute a Selected Tool Range:
# This example executes a range of tools 4 times, using different image references each #time.
tools = GetToolList()
for i in range(4):
SetValue('ImageRef.Value', i+1) # set the external scalar used as an image reference
tools.execute('Locate', 'ExportResults', 0) # execute tools named 'Locate' to #'ExportResults', ignoring manual tools
Example 2 – Change ToolList Execution Order:
# This example changes the execution order of tools by setting the 'next' attribute.
tools = GetToolList()
tools.execute('FindLine0', 'FindLine10')
tools.next = 'Blob2' # sets the next tool to be executed
Example 3 – Iterate Through TemplateFinder3 Tools:
# This example iterates through all TemplateFinder3 tools to classify the inspection status.
tools = GetToolList()
ok = 1
for i in range(tools.count):
tool = tools.tool(i)
if tool.type == 'TemplateFinder3' and not(tool.name == 'tf3'):
b0 = tool.getIntValue('Count') == 1 # check if the object is present
print(i+1, '-', tool.name, b0)
if not b0:
spb = SPB.CreateSpb(tool.config)
x, y = spb.getFloat('Center.X'), spb.getFloat('Center.Y')
DrawText('', x, y, tool.name, 'Red', 12)
if ok and not b0:
ok = 0
SetIntValue('Pass.Value', ok)
Example 4 – Access Tools Outside the Current Toolbox:
def browse(name):
print('--- tools in "{}" ---'.format(name))
tools = GetToolList(name)
try:
for i in range(tools.count):
t = tools.tool(i)
print(t.fullname, t.type)
except:
pass
browse(':') # root level
browse('tbox') # tools of toolbox 'tbox' at current level
browse('.') # tools at current level
browse('..') # tools at parent level
Conclusion: Scorpion Vision’s scripting capabilities provide an extensive toolset for developers to automate and fine-tune their vision systems. By mastering the ToolList
object and its associated methods, users can achieve easier maintenance, dynamic analysis, and sophisticated tool management to address complex vision inspection tasks. Remember that subtle changes via the script can dramatically change the behavior of the system, so it’s important to understand the impact of each operation within the context of the overall vision solution.